App - Chat
/chat is an example of the ultimate form of extensibility where instead of just being able to add Services, Methods and Plugins, etc. You can add your entire AppHost which Sharp Apps will use instead of its own. This vastly expands the use-cases that can be built with Sharp Apps as it gives you complete fine-grained control over how your App is configured.
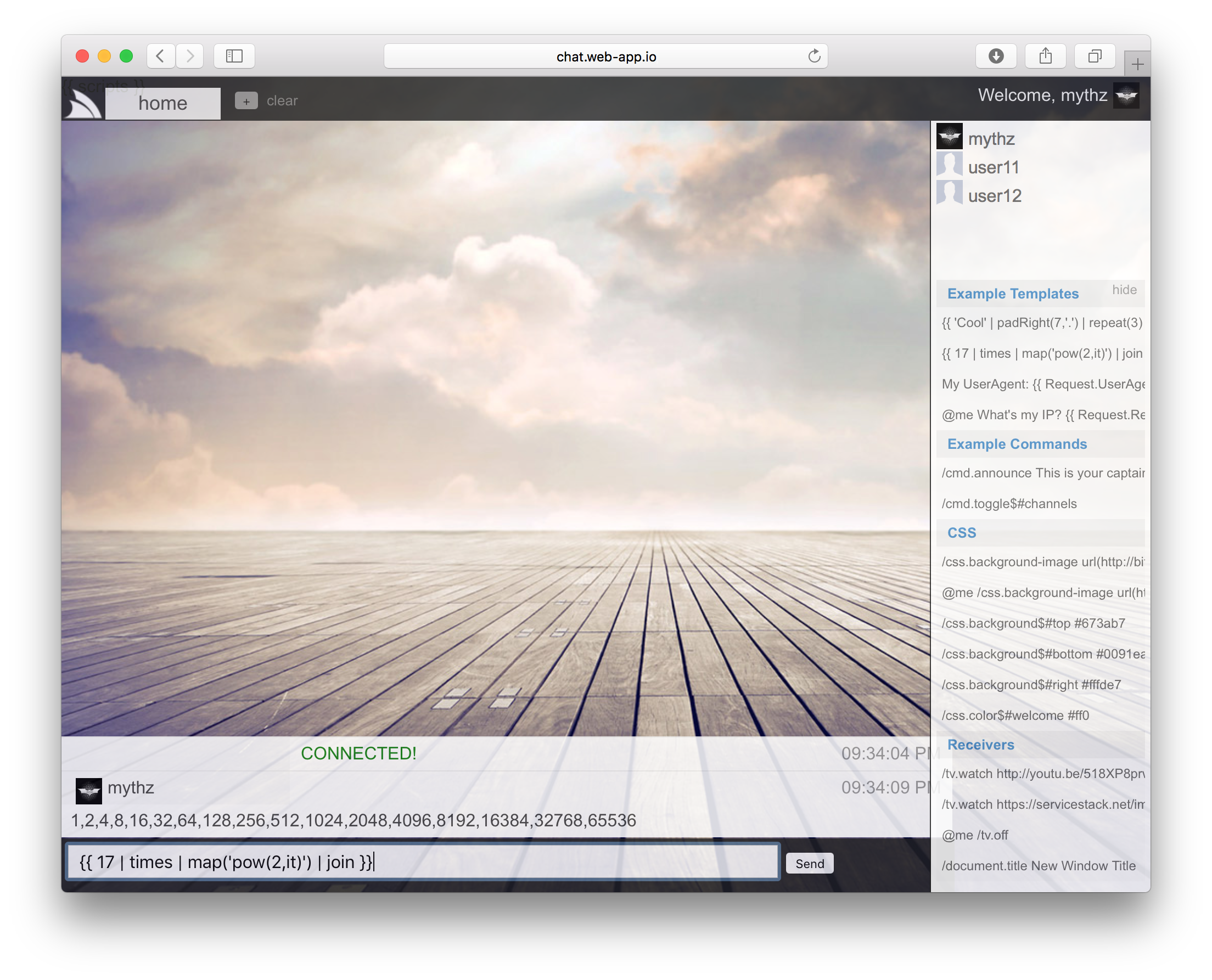
You can run this Gist Desktop App via URL Scheme from (Windows Desktop App):
Or via command-line:
$ app open chat
Cross platform (Default Browser):
$ x open chat
Develop back-end using .NET IDE's
For chat.web-app.io we've taken a copy of the existing .NET 5.0 Chat App and moved its C# code to /chat/src and its files to /chat where it can be developed like any other Web App except it utilizes the Chat AppHost and implementation in the SelfHost Chat App.
Customizations from the original .NET Core Chat implementation includes removing MVC and Razor dependencies and configuration, extracting its _layout.html and converting index.html to use #Script from its original default.cshtml. It's also been enhanced with the ability to evaluate scripts from the Chat window, as seen in the screenshot above.
Chat AppHost
public class Startup
{
public void Configure(IApplicationBuilder app)
{
var appSettings = new TextFileSettings("~/../app.settings".MapProjectPath());
app.UseServiceStack(new AppHost(appSettings));
}
}
public class AppHost : AppHostBase
{
public AppHost() : base("Chat Sharp App", typeof(ServerEventsServices).Assembly) {}
public AppHost(IAppSettings appSettings) : this() => AppSettings = appSettings;
public override void Configure(Container container)
{
Plugins.AddIfNotExists(new SharpPagesFeature()); //Already added if it's running as a Web App
Plugins.Add(new ServerEventsFeature());
SetConfig(new HostConfig
{
DefaultContentType = MimeTypes.Json,
AllowSessionIdsInHttpParams = true,
});
this.CustomErrorHttpHandlers.Remove(HttpStatusCode.Forbidden);
//Register all Authentication methods you want to enable for this web app.
Plugins.Add(new AuthFeature(
() => new AuthUserSession(),
new IAuthProvider[] {
new FacebookAuthProvider(AppSettings), //Sign-in with Facebook
}));
container.RegisterAutoWiredAs<MemoryChatHistory, IChatHistory>();
}
}
Reusing Web App's app.setting and files
One nice thing from being able to reuse existing AppHost's is being able to develop all back-end C# Services and Custom Filters as a stand-alone .NET Core Project where it's more productive with access to .NET IDE tooling and debugging.
To account for these 2 modes we use AddIfNotExists to only register the SharpPagesFeature plugin when running as a stand-alone App and add an additional constructor so it reuses the existing app.settings as its IAppSettings provider for is custom App configuration like OAuth App keys required for enabling Sign-In's via with Twitter, Facebook and GitHub when running on https://localhost:5001:
debug true
name Chat Sharp App
port 5001
oauth.RedirectUrl https://localhost:5001/
oauth.CallbackUrl https://localhost:5001/auth/{0}
oauth.facebook.Permissions email
oauth.facebook.AppId 531608123577340
oauth.facebook.AppSecret 9e1e6591a7f15cbc1b305729f4b14c0b
CefConfig { width:1150, height:1050 }
After the back-end has been implemented we can build and copy the compiled Chat.dll into the Chat's /plugins folder where we can take advantage of the improved development experience for rapidly developing its UI.